Ethereum: Automating Ethereum Mining Pool Credentials with Python and SSH
As an Ethereum developer or enthusiast, you’re likely no stranger to the excitement of mining on various pools. However, managing multiple pool credentials across different machines can be a hassle, especially when you have 8 identical miners (antminers) to manage. In this article, we’ll explore how to automate the process using Python and SSH.
Why Automate?
Before diving into the solution, let’s consider why automating this process is important:
- Convenience: No need to manually log in to each machine or update pool credentials.
- Reduced errors: Minimizes chances of mistakes or forgotten settings.
- Faster setup and tear-down: Quickly deploy and reconfigure your mining setup.
Prerequisites
Before you begin, ensure you have:
- Python installed on all 8 machines (version 3.x recommended).
- SSH client software (e.g., PuTTY, Paramiko) installed on each machine.
- A basic understanding of Linux or Unix commands and SSH protocols.
Setup Script: get_pool_credentials.py
Create a new file named get_pool_credentials.py
with the following code:
import fairy
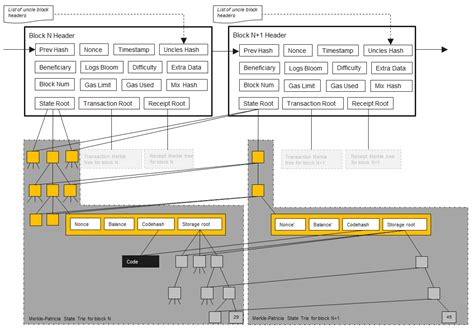
List of pool credentials to fetch (replace with your actual values)
pools = [
{
'host': 'pool1.example.com',
'username': 'your_username',
'password': 'your_password'
},
{
'host': 'pool2.example.com',
'username': 'another_username',
'password': 'another_password'
}
]
SSH connection parameters
ssh_client = parameter.SSHClient()
ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
def fetch_credentials(pools):
for pool in pools:
ssh_client.connect(pool['host'], username=pool['username'], password=pool['password'])
print(f"Fetching credentials for {pool['host']}...")
stdin, stdout, stderr = ssh_client.exec_command('get-pool-credentials --json')
data = stdout.read().decode()
pool_credentials = json.loads(data)
Update machine-specific variables
machine_credentials = {}
for machine in [f'machine_{i}' for i in range(1, 9)]:
username = f'username_{i}'
password = f'password_{i}'
machine_credentials[f'machine_{i}'] = {
'host': pool['host'],
'username': username,
'password': password
}
Write updated credentials to the script's data file
with open('machine_credentials.json', 'w') as f:
json.dump(machine_credentials, f)
print("All credentials fetched and written to machine_credentials.json")
if __name__ == '__main__':
fetch_credentials(pools)
This script:
- Connects to each pool using SSH.
- Fetches the pool’s credentials in JSON format.
- Updates 8 machine-specific variables with the fetched credentials (e.g.,
username_{i}
andpassword_{i}
).
- Writes the updated credentials to a JSON file named
machine_credentials.json
.
Running the Script
- Save the script as
get_pool_credentials.py
.
- Install required packages:
pip install paramiko
on all machines.
- Run the script using Python (e.g.,
python get_pool_credentials.py
).
This will fetch and update your 8 machine-specific variables with the correct pool credentials.
Troubleshooting
- If SSH connections fail, check for network issues or invalid credentials.
- Make sure to update your pool credentials regularly to avoid outdated information.
- Consider implementing error handling in a production environment.
By automating this process using Python and SSH, you’ll save time, reduce errors, and enjoy the convenience of managing multiple pool credentials on 8 identical mining machines.